Good contracts are on the heart of Web3 expertise, revolutionizing how transactions and agreements are dealt with. These self-executing items of code automate and implement the phrases of contracts with out the necessity for intermediaries. What’s extra, this modern expertise is paramount in creating decentralized functions (dapps), DeFi platforms, and different blockchain tasks. But, not everybody is aware of what good contracts are and how you can create them. Subsequently, on this article, we’ll dive into the fundamentals and present you how you can write a wise contract in Solidity!
Overview
We’ll kickstart in the present day’s article by leaping into the tutorial and displaying you how you can write a wise contract in Solidity. After the tutorial, we’ll cowl the intricacies of good contracts and the Solidity programming language. That stated, if you must refresh your reminiscence on both of those subjects earlier than going into the tutorial, we suggest beginning with the ”What’s a Good Contract?” part!
As soon as you know the way to jot down a wise contract in Solidity, you may wish to take your Web3 growth to the subsequent degree. And if that’s the case, then you definately’ll wish to discover Moralis!
Moralis supplies industry-leading Web3 APIs, making your work as a developer considerably simpler. As an illustration, with the NFT API, you may get a person NFT tokens listing, get ERC-721 on-chain metadata, and way more with a single line of code. As such, it has by no means been simpler to construct tasks centered round NFTs!
So, when you haven’t already, be sure to enroll with Moralis right away. You may create your Moralis account totally free and begin leveraging the true energy of blockchain expertise in the present day!
5-Step Tutorial: How you can Write a Good Contract in Solidity
Within the following sections, we’ll present you how you can write a wise contract in Solidity in 5 easy steps:
Step 1: Set Up RemixStep 2: Write the Good Contract Code in SolidityStep 3: Set Up MetaMaskStep 4: Get Testnet TokensStep 5: Compile and Deploy the Solidity Good Contract
The Solidity good contract you’ll write is basically easy, permitting you to retailer and retrieve an unsigned integer. Consequently, this tutorial is meant for builders with little to no expertise in Ethereum growth.
However, with no additional ado, let’s bounce into step one and present you how you can arrange Remix!
Step 1: Set Up Remix
You’ve many choices for writing a wise contract in Solidity. Nevertheless, to make this tutorial as beginner-friendly as doable, we’ll use Remix. Remix is a web-based built-in growth atmosphere (IDE) for creating EVM-compatible good contracts.
It’s easy and helps a number of options you want for this tutorial, together with the power to compile and deploy Solidity good contracts on the click on of some buttons. As such, let’s kick issues off by visiting the Remix web site.
Clicking on the hyperlink above takes you to the next web page, the place you’ll discover a workspace known as ”default_workspace”:
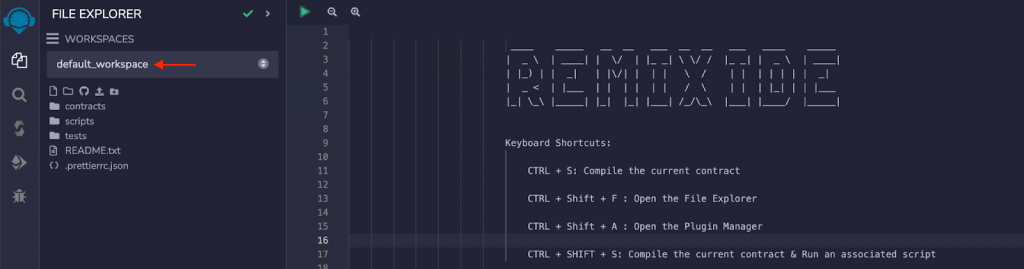
From there, open the ”contracts” folder, delete all of the default information, and create a brand new one known as ”Storage.sol”:
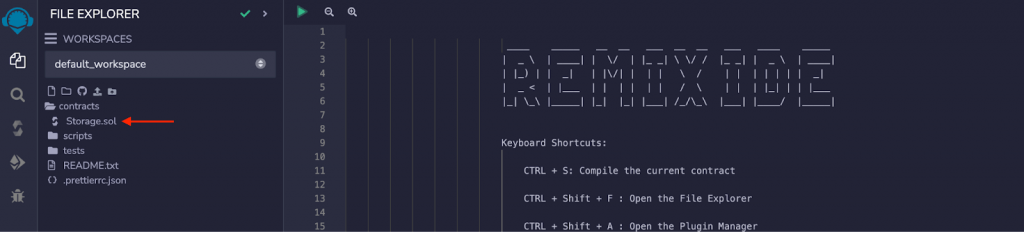
That’s it; you’re now prepared for the second step, the place we’ll present you how you can write the Solidity good contract!
Step 2: Write the Good Contract Code in Solidity
For the second step, we’ll present you how you can write the good contract code in Solidity. As such, go forward and open the ”Storage.sol” file and enter the next code:
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.2 <0.9.0;
contract Storage {
uint knowledge;
perform set(uint x) public {
knowledge = x;
}
perform get() public view returns (uint) {
return knowledge;
}
}
The code above is the whole contract, and we’ll now break down every row, ranging from the highest!
On the preliminary line, we specify the SPDX license sort. Each time the supply code of a contract is made publicly out there, these licenses may also help keep away from and resolve copyright points:// SPDX-License-Identifier: MIT The third line of the code declares which Solidity compiler you wish to use. On this case, we’re utilizing any model equal to or between 0.8.2 and 0.9.0: pragma solidity >=0.8.2 <0.9.0; On the fifth line, we declare the contract, and on this case, we title it Storage. It’s frequent observe to make use of the identical title for the file and contract:contract Storage The sixth line declares an unsigned integer (uint) known as knowledge. That is the variable that can retailer the information you assign when executing the features of the contract: uint knowledge; On traces seven to 9, we declare the set() perform. This perform takes a uint variable as a parameter and assigns it to knowledge. Moreover, the key phrase public ensures that anybody can name the set() perform: perform set(uint x) public {
knowledge = x;
} Within the final couple of traces, we add the get() perform that returns the worth of the information variable. The view key phrase tells the Solidity compiler it’s a read-only perform, and the returns (uint) half specifies that the perform is to return a uint variable:perform get() public view returns (uint) {
return knowledge;
}
That’s it; you now know how you can write a wise contract in Solidity!
Within the subsequent step, we’ll present you how you can arrange MetaMask, which you’ll have to compile and deploy the contract within the tutorial’s last half!
Step 3: Set Up MetaMask
Now that you just’re executed writing the code in your Solidity good contract, the subsequent factor you’ll want is a Web3 pockets. And for this tutorial, we’ll be utilizing MetaMask. As such, when you haven’t already, head on over to “metamask.io” and hit the ”Obtain” button on the backside left:
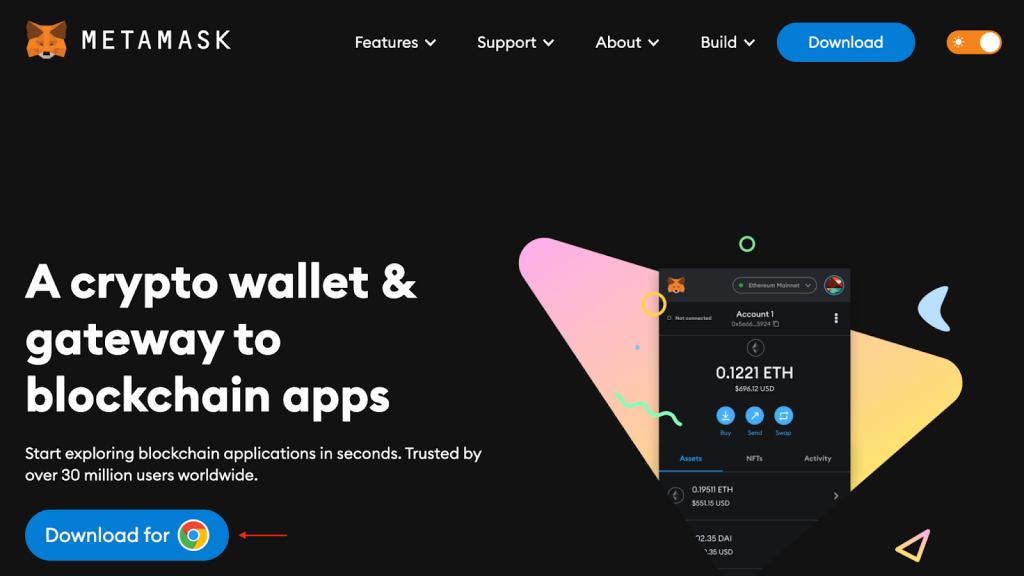
Subsequent, add MetaMask as a browser extension:
When you add MetaMask to your browser, you’ll be taken to the next web page, the place you may both arrange a brand new pockets or import an present one:
After you create a brand new or import a pockets, it is best to end up on a web page trying one thing like this:
Along with establishing a MetaMask account, you should add Ethereum’s Sepolia testnet to your pockets. The explanation for doing that is that we don’t wish to instantly decide to the Ethereum mainnet. As an alternative, it is best to deploy your good contract to Sepolia so you may take a look at it in a protected and safe atmosphere.
So as to add and swap to the Sepolia testnet, go forward and hit the networks drop-down menu on the prime proper of your MetaMask interface, click on on the ”Present take a look at networks” button, and choose Sepolia:
Afterwards, it ought to now look one thing like this:
Step 4: Get Testnet Tokens
Deploying a wise contract to a blockchain requires a community transaction, that means you should pay a gasoline price. Consequently, to be able to deploy your Solidity good contract, you must load up on some Sepolia ETH. Happily, since Sepolia is a testnet, you may leverage a faucet to get free tokens. And the simplest option to discover a legit faucet is to go to the Moralis testnet taps web page!
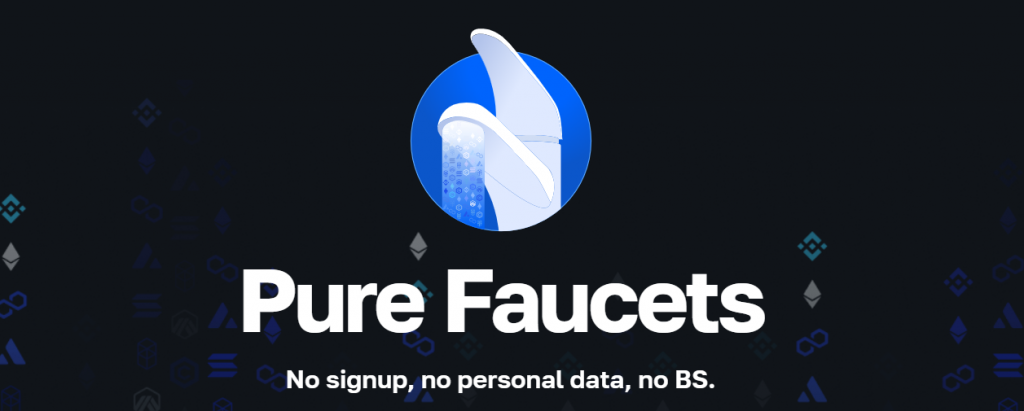
The Moralis taps web page comprises a curated listing of free testnet taps you may belief. No signups are required. No private knowledge is required. And no BS!
To entry the Sepolia testnet faucet, merely head on over to the taps web page, scroll down, and click on on ”Strive Now” for the Sepolia choice:
Clicking this takes you to the next web page the place you must enter your MetaMask pockets tackle and click on on ”Begin Mining”:
When you click on on this button, you’ll begin to mine Sepolia testnet ETH. It ought to look one thing like this:
After a couple of minutes, you may click on on the ”Cease Mining” button, permitting you to assert your tokens. As quickly as you declare them, the stability in your MetaMask pockets ought to replace:
From right here, now you can use these testnet tokens to pay for transactions on the Sepolia testnet. This implies you’re now prepared for the ultimate step, the place we’ll present you how you can compile and deploy your Solidity good contract!
Step 5: Compile and Deploy the Solidity Good Contract
For the ultimate step, you initially have to compile the contract. To take action, return to Remix, navigate to the ”Solidity compiler” tab, and hit the ”Compile…” button:
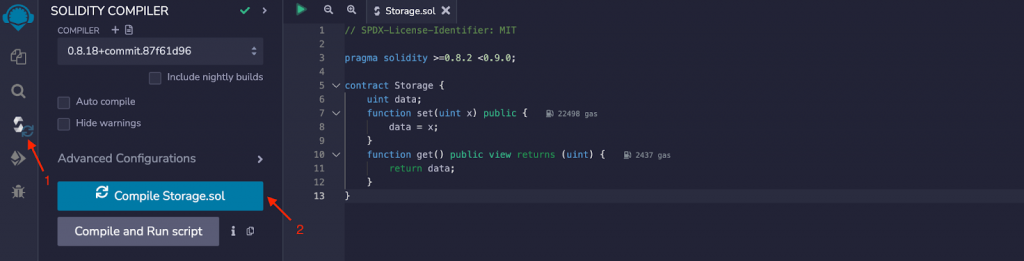
After compiling the contract, you’re now able to deploy it. As such, go to the ”Deploy & Run transactions” tab:
Right here, the very first thing you must do is choose ”Injected Supplier – MetaMask” for the atmosphere. Clicking on this button will immediate your MetaMask pockets, permitting you to signal a message:
After deciding on the atmosphere, you may hit the ”Deploy” button. Doing so will, as soon as once more, immediate your MetaMask pockets, the place you must signal and pay for the transaction utilizing your Sepolia testnet tokens:
When you affirm the transaction, it would take a while to get authorised. However when it does, you’ll see a message within the Remix console:
Now, it is best to have the ability to view and take a look at the features of your contract beneath the ”Deployed Contracts” part:
That’s it; you will have now efficiently created and deployed a easy Solidity good contract to the Sepolia testnet!
What’s a Good Contract?
Briefly, a wise contract is a program saved on a blockchain community that executes each time predetermined circumstances are met. These contracts are normally used to automate the execution of agreements the place individuals will be sure of the outcomes. Moreover, since code handles the method, there’s no want for any middleman’s involvement.
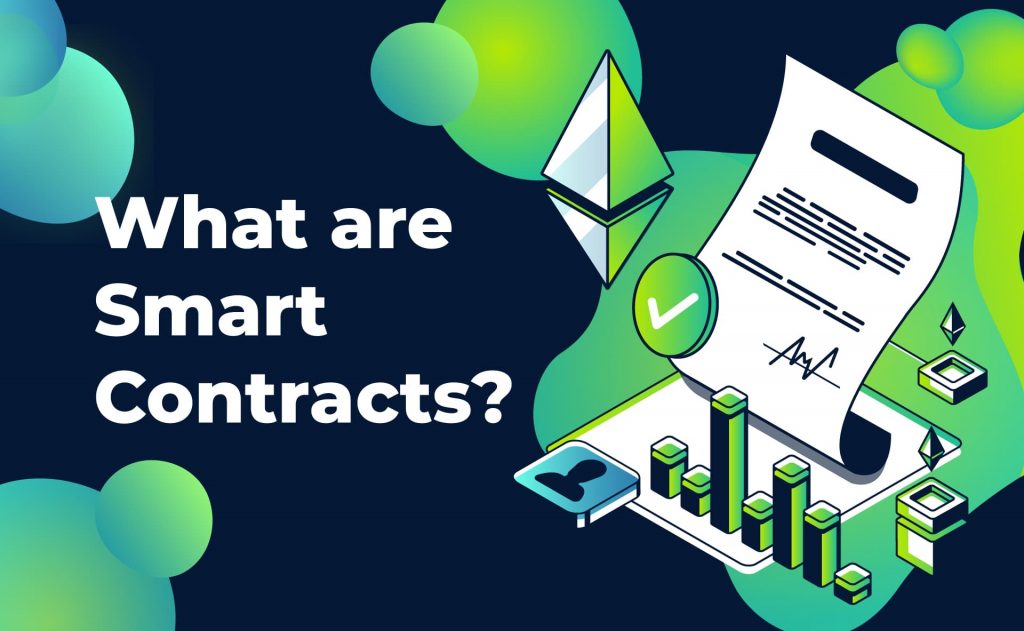
Good contracts usually encompass two components: knowledge and a set of code. The information is the contract’s state; in the meantime, the code makes up the features of the contract. These two components of every contract reside at their very own tackle on a blockchain community.
Moreover, all good contracts on the Ethereum community are a sort of account. Because of this every good contract has the power to make transactions and maintain a stability. Nevertheless, they differ from common accounts in that no person controls them. As an alternative, the contract/account is managed by the code throughout the contract itself.
Since good contracts are accounts, it’s doable for you as a person to work together with them. As an illustration, you may submit a transaction, and in return, the contract executes the features throughout the code. Consequently, the contract basically provides a algorithm after which enforces them when the predetermined circumstances are met.
In case you’d prefer to be taught extra about this, please take a look at our article explaining Web3 contracts in additional element!
What’s Solidity?
Solidity is the most well-liked programming language for good contract growth. It’s an object-oriented, high-level language Web3 builders use to jot down EVM-compatible good contracts.
It’s a curly bracket language with many influences from different programming languages, equivalent to C++ and JavaScript. Consequently, in case you have prior information of, as an illustration, JavaScript, you’ll have an edge in selecting up Solidity sooner.
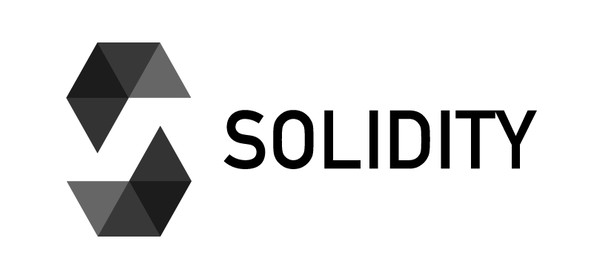
Moreover, Solidity is also known as the primary contract-oriented language. Because of this it’s tailored for creating good contracts. Consequently, it’s outfitted with many built-in instructions to allow a extra accessible blockchain growth expertise. As an illustration, builders can simply entry issues like addresses or timestamps of a selected block.
When you’re executed writing a Solidity good contract, it’s compiled to EVM bytecode. This makes these packages appropriate with any EVM blockchain. As such, Solidity is a programming language that can be utilized to jot down good contracts for a number of blockchain networks, together with Ethereum, Polygon, BNB Good Chain, and lots of others.
However, this solely covers the fundamentals of the programming language; when you’d prefer to be taught extra, take a look at our in-depth breakdown of Solidity.
Conclusion: How you can Write a Good Contract in Solidity
In in the present day’s article, we confirmed you how you can write a wise contract in Solidity in 5 easy steps:
Step 1: Set Up RemixStep 2: Write the Good Contract Code in SolidityStep 3: Set Up MetaMaskStep 4: Get Testnet TokensStep 5: Compile and Deploy the Solidity Good Contract
So, in case you have adopted alongside this far, you know the way to jot down a easy good contract in Solidity!
Now, when you’d prefer to take your Web3 growth to the subsequent degree, you’ll additionally wish to take a look at Moralis additional. Moralis supplies a set of industry-leading Web3 APIs, together with the Streams API, Value API, Pockets API, and lots of others! Moreover, when you want some inspiration on what’s doable to construct utilizing Moralis, take a look at Moralis Cash!
With the instruments talked about above, you may create Web3 tasks shortly and effortlessly, saving you and your growth staff an abundance of growth sources and time. Simply take a look at some testimonials of completely happy prospects already leveraging Moralis to chop prices:
So, take this chance and enroll with Moralis in the present day. You may arrange your Moralis account fully totally free and begin constructing tasks sooner and extra successfully!